Nighshark: Create your own custom function.
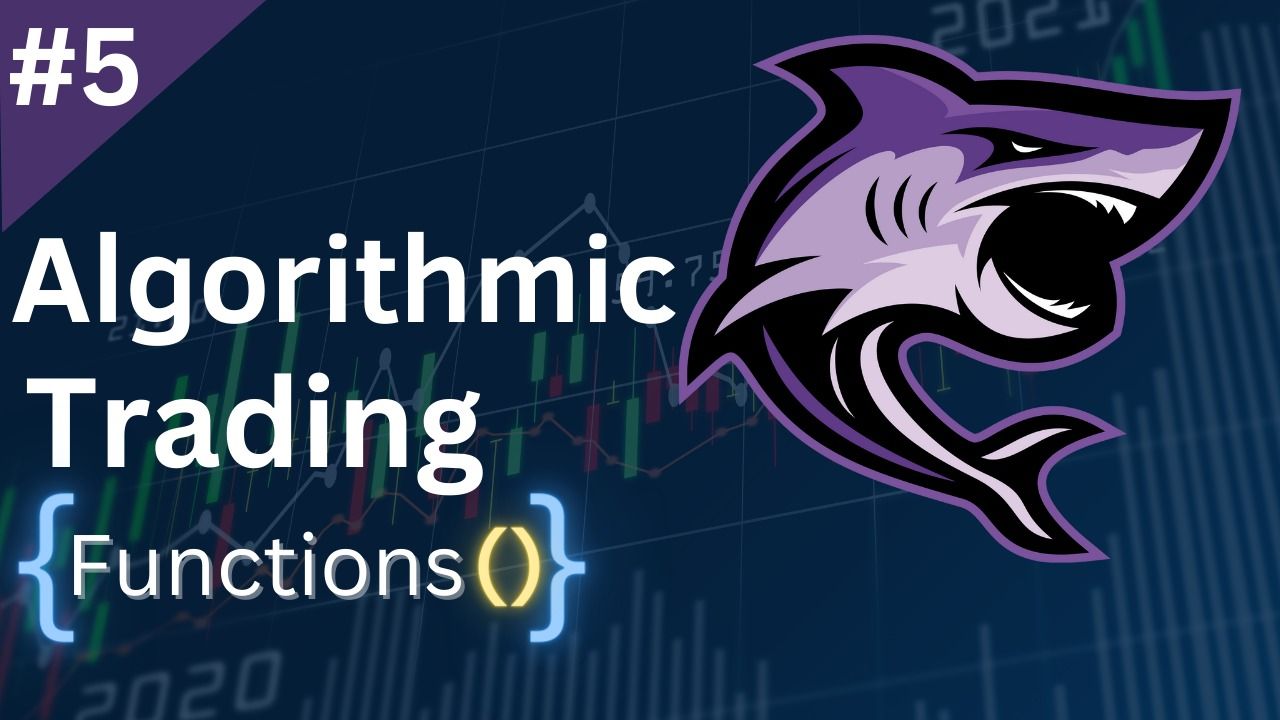
Introduction
NightShark, a robust platform for algo-trading, offers a wide array of built-in functionalities. However, one of its most powerful features is the ability to declare custom functions. This enables traders to encapsulate specific actions or conditions, thereby making trading algorithms more modular and easier to manage. In this blog post, we'll delve into the concept of declaring and implementing custom functions in NightShark, focusing on a profit and loss management example.
What Are Functions?
In programming, a function is a block of reusable code designed to perform a specific task. Functions can take inputs, process them, and optionally return an output. They are crucial for avoiding code repetition and for breaking down complex algorithms into more manageable parts.
Why Declare Custom Functions?
- Code Reusability: Functions can be invoked multiple times, reducing code duplication.
- Modularity: Functions allow you to segment your code into logical blocks, making it easier to debug and maintain.
- Readability: Well-named functions can make your code more self-explanatory, thereby improving readability.
How to Declare a Function in NightShark
Declaring a function in NightShark is straightforward. Here's a simple example where a function named CheckPnL
is defined to manage profit and loss:
; Function to check Profit and Loss
CheckPnL() {
loop {
read_areas()
if (toNumber(area[2]) > 100 || toNumber(area[2]) < 50)
break
}
}
In this example, the function CheckPnL
is declared to loop continuously, reading the value of area[2]
. If this value is greater than 100 (indicating a profit of 100) or less than 50 (indicating a loss of 50), the loop breaks, effectively stopping the trading algorithm.
Implementing the Function
Once a function is declared, it can be implemented in your trading algorithm. Here's how you could use the CheckPnL
function:
loop {
// Your trading logic here, e.g., buying or selling stocks
// ...
// Check Profit and Loss
CheckPnL()
}
In this loop, your trading logic would be implemented, and then the CheckPnL()
function is called. If the profit reaches 100 or the loss hits 50, the CheckPnL()
function will break its loop, allowing you to take appropriate actions.
Conclusion
Declaring custom functions in NightShark offers a way to make your algo-trading strategies more efficient and manageable. By encapsulating specific actions or conditions into functions, you can create modular, reusable, and readable code. The CheckPnL
function serves as a powerful example of how custom functions can be used to manage profit and loss effectively. With custom functions, you're not just writing code; you're crafting a well-organized, efficient trading strategy.
Happy Trading!